About this codelab
HUAWEI Ads Publisher Service utilizes Huawei\'s vast user base and extensive data capabilities to deliver targeted, high quality ad content to users. With this service, your app will be able to generate revenues while bringing your users content which is relevant to them.
We offer a range of ad formats so you can choose whichever that suits your app best. Currently, you can integrate banner, native, rewarded, interstitial, splash, and roll ads, and we'll be launching even more formats in the future.
Use the HUAWEI Ads SDK to quickly integrate HUAWEI Ads into your app. Once you have chosen an ad format, you are ready to start bringing in revenue using our high-quality advertising service
What You Will Create
In this codelab, you will use the demo project that has been created for you to experience Ads Kit APIs. Through the demo project, you will experience developing an all ad types and features with;
- How to developt app with Kotlin lanugage
- How to use Huaewi Ads Kit SDK
- How to use SplashAd
- How to use BannerAd
- How to use InterstialAd
- How to use RewardAd
- How to use NativeAd
- How to use InStreamRollAd
What You Will Learn
In this codelab, you will learn how to:
- Initialize all ad types of Ads Kit SDK
- Integrate and use abilities of Ads Kit SDK
Hardware Requirements
- One PC with Android Studio installed
- One mobile phone with a USB data cable for running developed apps
Software Requirements
- Android Studio 3.x or later version(Download)
- Java JDK 1.8 or later version(Download)
- EMUI 5.0 or later
- HMS Core (APK) 5.0.1.300 or later version
- Android 4.4 or later
Required Knowledge
- Android development basics
- Basic Kotlin language knowledge
Before you get started, complete the following preparations:
- Create an app in AppGallery Connect.
- Create an Android Studio project.
- Generate a signing certificate.
- Generate a signing certificate fingerprint.
- Configure the signing certificate fingerprint.
- Add the app package name and save the configuration file.
- Configure the Maven repository address and AppGallery Connect gradle plug-in.
- Configure the signature file in Android Studio.
For details, please refer to Preparations for Integrating HUAWEI HMS.
You can download the codelab project from: https://github.com/lookub/AdsByHuaweiCodelab.git
Creating a Project
Step 1: Start Android Studio.
Step 2: Choose File > Open, go to the directory where the sample project is decompressed, and click OK.
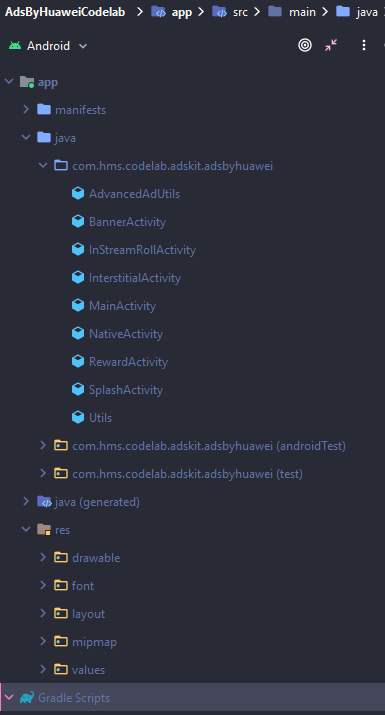
Step 3: Configure the AppGallery Connect plug-in, Maven repository address, build dependencies, obfuscation scripts, and permissions. (These items have been configured in the sample code. If any of them does not meet your requirements, change it in your own project.)
1. Configure the Maven repository address and AppGallery Connect plug-in in the project's build.gradle file.
- Go to allprojects > repositories and configure the Maven repository address for the HMS Core SDK.
allprojects { repositories { maven { url 'https://developer.huawei.com/repo/' } ... } }
- Go to buildscript > repositories and configure the Maven repository address for the HMS Core SDK.
buildscript { repositories { maven {url 'https://developer.huawei.com/repo/'} ... } ... }
2. Configure the dependency package in the app's build.gradle file.
- Add a dependency package to the dependencies section in the build.gradle file.
dependencies { ... // Ads Kit SDK implementation 'com.huawei.hms:ads-lite:13.4.36.301' ... }
For Ads Kit SDK, please refer to latest version.
3. Configure obfuscation scripts.
- Configure the following information in the app/proguard-rules.pro file:
-ignorewarnings -keep class com.huawei.openalliance.ad.** { *; } -keep class com.huawei.hms.ads.** { *; }
4. Configure permissions in the AndroidManifest.xml file.
<uses-permission android:name="android.permission.INTERNET"/>
// Add this congiguration to in application scope on manigers file.
//For To allow HTTP network requests on devices with targetSdkVersion 28 or later
android:usesCleartextTraffic="true"
Step 4: In the Android Studio window, choose File > Sync Project with Gradle Files to synchronize the project.
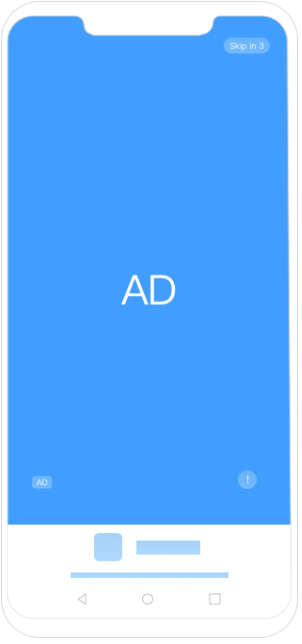
0. Locate following line to create Initialize the HUAWEI Ads SDK! in onCreate()
// TODO : Initialize the HUAWEI Ads SDK.
HwAds.init(this)
1. Locate following line to create SplashView, SplashAdDisplayListener and SplashAdLoadListener
// TODO : create splashView
private lateinit var splashView: SplashView
// TODO : create listeners
private lateinit var adDisplayListener: SplashAdDisplayListener
private lateinit var splashAdLoadListener: SplashAdLoadListener
2. Locate following line to invoke Splash Ad Listeners create methods
// TODO : create Listeners
createAdLoadListener()
createAdDisplayListener()
2.1. Locate following line to create SplashAdLoadListener
// TODO : create createAdLoadListener
private fun createAdLoadListener() {
splashAdLoadListener = object : SplashAdLoadListener() {
override fun onAdLoaded() {
Log.i(TAG, "SplashAdLoadListener onAdLoaded.")
}
override fun onAdFailedToLoad(errorCode: Int) {
val errorMessage = AdvancedAdUtils.getDetailsFromErrorCode(errorCode)
Log.e(TAG, "SplashAdLoadListener.onAdFailedToLoad() with errorMessage $errorMessage" )
// jump from splahScreen to MainActivity ( call startActivity )
jump()
}
override fun onAdDismissed() {
Log.i(TAG, "SplashAdLoadListener onAdDismissed.")
// jump from splahScreen to MainActivity ( call startActivity )
jump()
}
}
}
2.2. Locate following line to create SplashAdDisplayListener
// TODO : create createAdDisplayListener
private fun createAdDisplayListener() {
adDisplayListener = object : SplashAdDisplayListener() {
override fun onAdClick() {
Log.i(TAG, "SplashAdDisplayListener onAdShowed.");
}
override fun onAdShowed() {
Log.i(TAG, "SplashAdDisplayListener onAdClick.")
}
}
}
3. Locate following line to Initialize SplashView and load Ad
// TODO : load Ad
private fun loadAd() {
val adParam = AdParam.Builder().build()
// Obtain SplashView.
splashView = findViewById(R.id.splash_ad_view)
splashView.setAdDisplayListener(adDisplayListener)
// Set a logo image.
splashView.setLogoResId(R.drawable.icon_ads)
// Set logo description.
splashView.setMediaNameResId(R.string.app_name)
// Set the audio focus type for a video splash ad.
splashView.setAudioFocusType(AudioFocusType.NOT_GAIN_AUDIO_FOCUS_WHEN_MUTE)
// Load the ad. AD_ID indicates the ad slot ID.
splashView.load(
getString(R.string.ad_id_splash),
ActivityInfo.SCREEN_ORIENTATION_PORTRAIT,
adParam,
splashAdLoadListener
)
}
4. Locate following line to add SplashView to XML layout file.
// <!-- TODO : add SplashView XML layout -->
<com.huawei.hms.ads.splash.SplashView
android:id="@+id/splash_ad_view"
android:layout_width="0dp"
android:layout_height="0dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
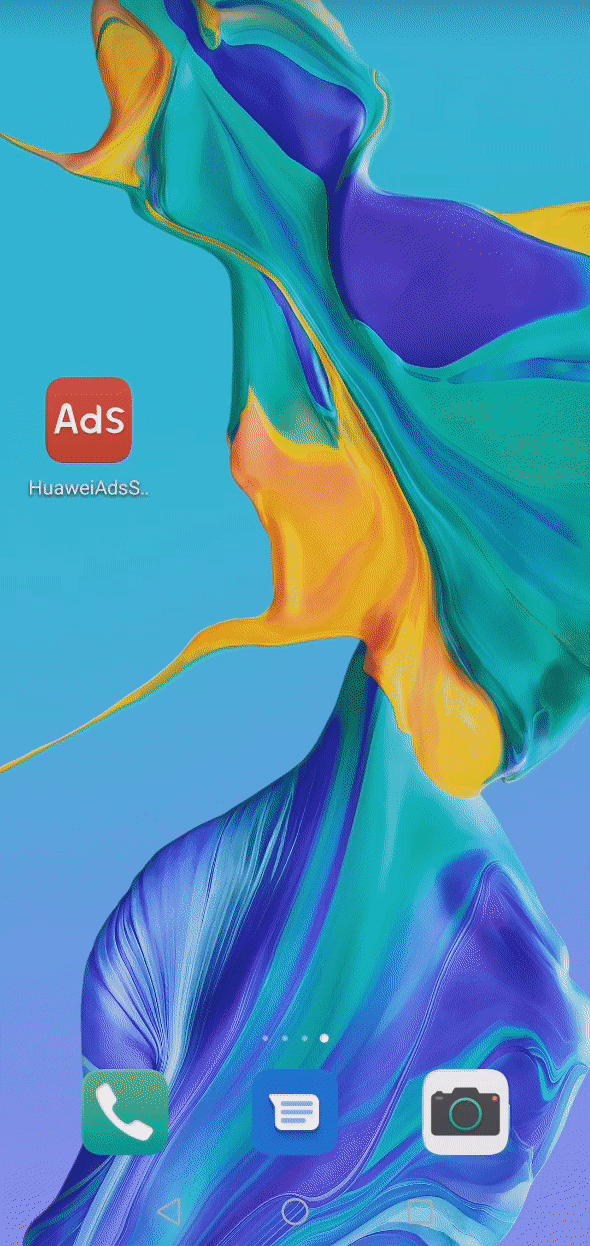
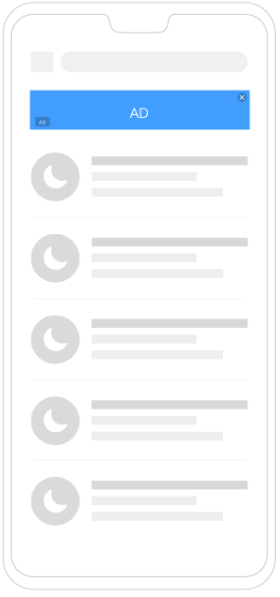
1. Locate following line to ad BannerView and FrameLayout to XML layout file.
// <!-- TODO : add BannerView and FrameLayout XML layout -->
<FrameLayout
android:id="@+id/ad_frame"
android:layout_width="match_parent"
android:layout_height="wrap_content"
app:layout_constraintBottom_toTopOf="@id/hw_banner_view"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent" />
<com.huawei.hms.ads.banner.BannerView
android:id="@+id/hw_banner_view"
android:layout_width="match_parent"
android:layout_height="wrap_content"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
hwads:adId="testw6vs28auh3"
hwads:bannerSize="BANNER_SIZE_360_57" />
2. Locate following line to create BannerView, FrameLayout and AdListener
// TODO : create BannerView
private lateinit var bannerView: BannerView
// TODO : create FrameLayout
private lateinit var adFrameLayout: FrameLayout
// TODO : create listeners
private lateinit var adListener: AdListener
3. Locate following line to Initialize BannerView, FrameLayout
// TODO : initialize FrameLayout
adFrameLayout = findViewById(R.id.ad_frame)
// TODO : initialize BannerView
bannerView = findViewById(R.id.hw_banner_view)
4. Locate following line to Initialize AdListener
// TODO : initialize AdListener
adListener = object : AdListener() {
override fun onAdLoaded() {
super.onAdLoaded()
Log.d(TAG,"adListener.onAdLoaded")
}
override fun onAdFailed(errorCode: Int) {
val errorMessage = AdvancedAdUtils.getDetailsFromErrorCode(errorCode)
Log.e(TAG,"adListener.onAdFailed() with errorMessage $errorMessage")
}
override fun onAdClosed() {
super.onAdClosed()
Log.d(TAG,"adListener.onAdClosed")
}
override fun onAdClicked() {
Log.d(TAG,"adListener.onAdClicked")
super.onAdClicked()
}
override fun onAdOpened() {
Log.d(TAG,"adListener.onAdOpened")
super.onAdOpened()
}
}
5. Locate following line to invoke Splash Ad Listeners create methods
// TODO : customize and load BannerAd
if (bannerView != null) {
adFrameLayout.removeView(bannerView)
bannerView.destroy()
}
bannerView = BannerView(this)
bannerView.adId = getString(R.string.ad_id_banner)
bannerView.setBackgroundColor(Color.BLUE)
bannerView.bannerAdSize = BannerAdSize.BANNER_SIZE_360_57
bannerView.setBannerRefresh(5)
bannerView.adListener = adListener
bannerView.loadAd(AdParam.Builder().build())
adFrameLayout.addView(bannerView)
// can customize ad RequestOptions parameters
// Advanced Settings
4. The following table lists the standard Banner Ad Sizes :
Type | Size in dp (W x H) | Description |
BANNER_SIZE_320_50 | 320 x 50 | Common banner ads, applicable to phones. |
BANNER_SIZE_320_100 | 320 x 100 | Large banner ads, applicable to phones. |
BANNER_SIZE_300_250 | 300 x 250 | Medium rectangular banner ads, applicable to phones. |
BANNER_SIZE_360_57 | 360 x 57 | Common banner ads, applicable to 1080 x 170 px ad assets. |
BANNER_SIZE_360_144 | 360 x 144 | Large banner ads, applicable to 1080 x 432 px ad assets.. |
BANNER_SIZE_SMART | Screen width x 32|50|90 | Adaptive banner ads (whose size is automatically adjusted based on the aspect ratios of devices), applicable to phones. |
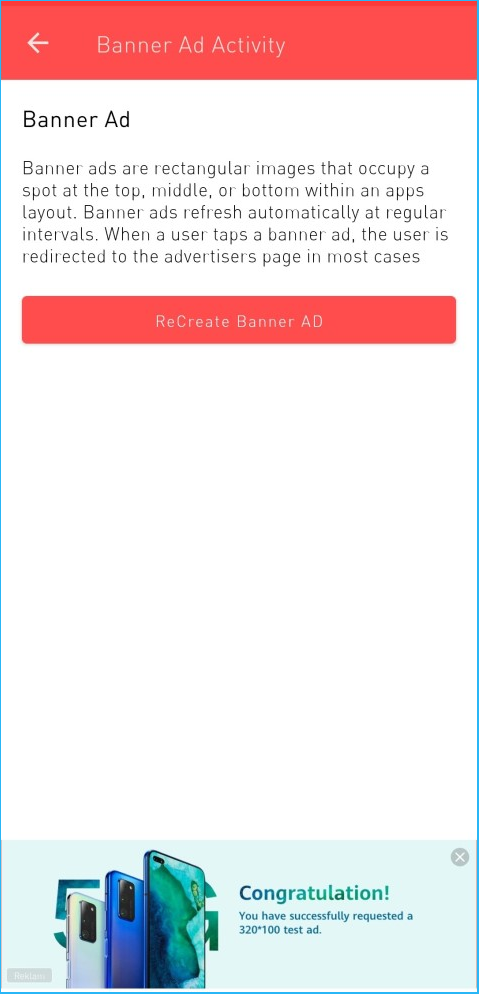
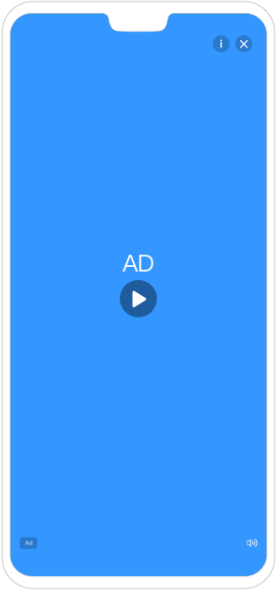
1. Locate following line to create InterstitialAd and AdListener
// TODO : create listeners
private lateinit var adListener : AdListener
// TODO : create InterstitialAd
private lateinit var interstitialAd: InterstitialAd
2. Locate following line to Initialize BannerView, FrameLayout
// TODO : initialize FrameLayout
adFrameLayout = findViewById(R.id.ad_frame)
// TODO : initialize BannerView
bannerView = findViewById(R.id.hw_banner_view)
3. Locate following line to Initialize AdListener
// TODO : initialize AdListener
adListener = object : AdListener() {
override fun onAdLoaded() {
super.onAdLoaded()
Log.d(TAG, "adListener.onAdLoaded")
// TODO : Display an interstitial ad when its loaded
showInterstitial()
}
override fun onAdFailed(errorCode: Int) {
Log.e(TAG,"adListener.onAdFailed() with errorCode $errorCode")
}
override fun onAdClosed() {
super.onAdClosed()
Log.d(TAG, "adListener.onAdClosed")
}
override fun onAdClicked() {
Log.d(TAG, "adListener.onAdClicked")
super.onAdClicked()
}
override fun onAdOpened() {
Log.d(TAG, "adListener.onAdOpened")
super.onAdOpened()
}
}
}
4. Locate following line to Initialize InterstitialAd
// TODO : initialize InterstitialAd
interstitialAd = InterstitialAd(this)
interstitialAd.adId = R.string.ad_id_interstitial_video
interstitialAd.adListener = adListener
val adParam = AdParam.Builder().build()
interstitialAd.loadAd(adParam)
5. Locate following line to Display InterstitialAd
// TODO : Display an interstitial ad when its loaded
if (interstitialAd != null && interstitialAd.isLoaded) {
interstitialAd.show()
} else {
Log.d(TAG, "interstitialAd is NULL or did not Loaded")
}
6. The following table lists the standard Banner Ad Sizes :
Display Form | Screen Orientation | Dimensions | Test Ad Slot ID |
Image | Portrait | 1080 x 1620 | teste9ih9j0rc3 |
Video | Portrait | 720 x 1080 | testb4znbuh3n2 |
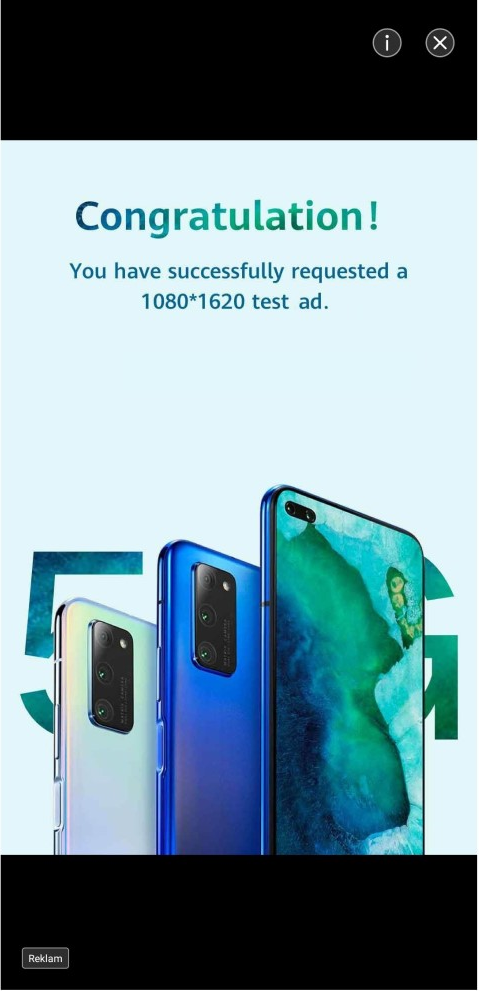
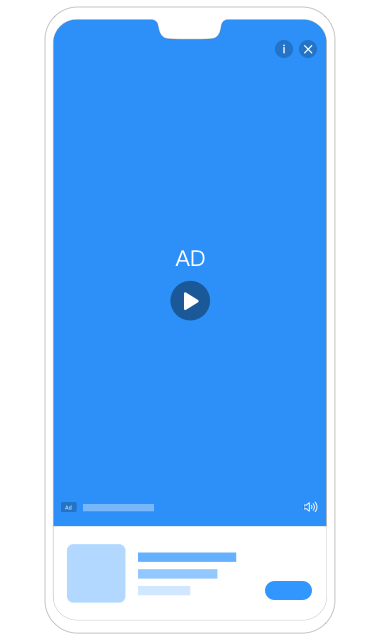
1. Locate following line to create RewardAd and AdStatusListener
// TODO : create RewardAd
private lateinit var rewardedAd: RewardAd
// TODO : create adStatus listeners
private lateinit var rewardAdListener: RewardAdStatusListener
2. Locate following line to Initialize AdStatusListener
// TODO : create adStatus listener
rewardAdListener = object : RewardAdStatusListener() {
override fun onRewardAdFailedToShow(errorCode: Int) {
Log.e(TAG,"RewardAdStatusListener.onRewardAdFailedToShow() with errorCode $errorCode")
}
override fun onRewardAdClosed() {
Log.d(TAG,"RewardAdStatusListener.onRewardAdClosed() Ad will reCreate")
// TODO : call initialize RewardAd
createAndLoadRewardAd()
}
override fun onRewardAdOpened() {
Log.e(TAG,"RewardAdStatusListener.onRewardAdOpened")
}
override fun onRewarded(reward: Reward) {
// You are advised to grant a reward immediately and at the same time, check whether the reward
// takes effect on the server. If no reward information is configured, grant a reward based on the
// actual scenario.
// You are advised to grant a reward immediately and at the same time, check whether the reward
// takes effect on the server. If no reward information is configured, grant a reward based on the
// actual scenario.
val addScore = if (reward.amount == 0) defaultScore else reward.amount
Log.d(TAG,"onRewarded() : reward.getAmount() you can use addScore : $addScore")
// TODO : optional : call initialize RewardAd for reInitialize for after usage
createAndLoadRewardAd()
}
}
3. Locate following line to Initialize RewardAd
// TODO : initialize RewardAd
rewardedAd = RewardAd(this@RewardActivity, getString(R.string.ad_id_reward))
val rewardAdLoadListener: RewardAdLoadListener = object : RewardAdLoadListener() {
override fun onRewardAdFailedToLoad(errorCode: Int) {
Log.e(TAG,"RewardAdStatusListener.onRewardAdFailedToLoad() with errorCode $errorCode")
}
override fun onRewardedLoaded() {
Log.d(TAG, "rewardAdLoadListener.onRewardedLoaded.")
}
}
rewardedAd.loadAd(AdParam.Builder().build(), rewardAdLoadListener)
4. Locate following line to Display RewardAd
// TODO : display RewardAd
if (rewardedAd.isLoaded) {
rewardedAd.show(this@RewardActivity, rewardAdListener)
}
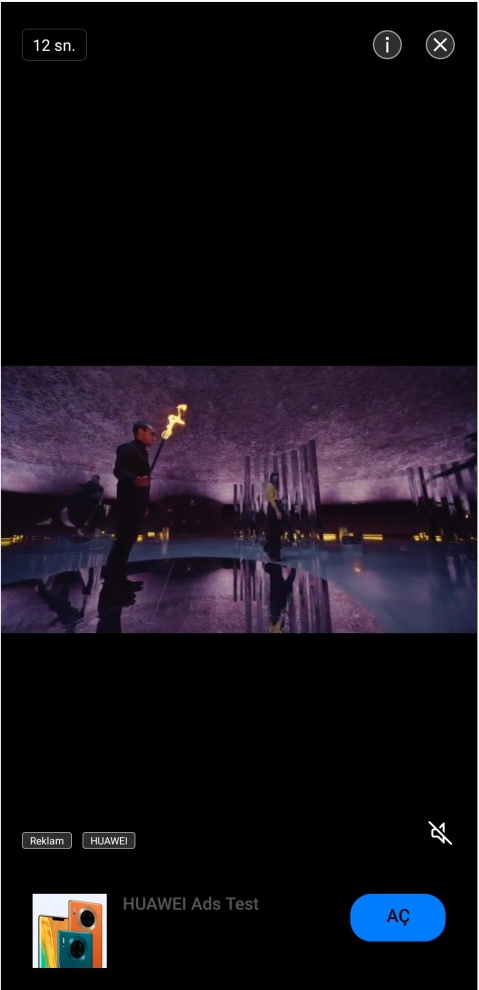
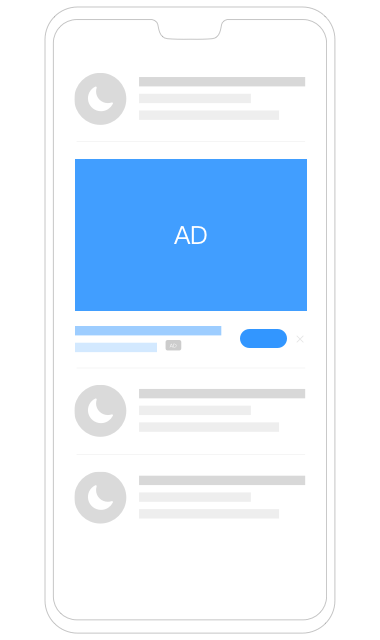
0. Locate following line to ad Native Ad template layouts layout include element to XML layout file.
// <!-- add native_video_template layout include element -->
<include
android:id="@+id/native_ad_video"
layout="@layout/native_video_template"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginBottom="40dp" />
// <!-- add native_small_template layout include element -->
<include
android:id="@+id/native_ad_small"
layout="@layout/native_small_template"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_marginBottom="40dp" />
// <!-- add native_large_template layout include element -->
<include
android:id="@+id/native_ad_large"
layout="@layout/native_large_template"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:layout_marginBottom="40dp" />
1. Locate following line to find these layout xml files.( will also be in project layout files
2. Locate following line to create NativeView whatever type you want, and create VideoLifecycleListener for videoNativeView
// TODO : create NativeView for smallNativeView
private lateinit var smallNativeView: NativeView
// TODO : create NativeView for largeNativeView
private lateinit var largeNativeView: NativeView
// TODO : create NativeView for videoNativeView
private lateinit var videoNativeView: NativeView
// TODO : create VideoLifecycleListener for videoNativeView
private lateinit var videoLifecycleListener: VideoLifecycleListener
// TODO : create AdListener
private lateinit var adListener: AdListener
3. Locate following line to Initialize NativeView whatever type you want
// TODO : Initialize NativeView
smallNativeView = findViewById(R.id.native_ad_small)
largeNativeView = findViewById(R.id.native_ad_large)
videoNativeView = findViewById(R.id.native_ad_video)
4. Locate following line to call AdListeners
// TODO : Initialize AdListener
createNativeAdListener()
// TODO : call createVideoLifecycleListener
createVideoLifecycleListener()
4.1. Locate following line to Initialize AdListener
// TODO : Initialize createAdListener
adListener = object : AdListener() {
override fun onAdLoaded() {
super.onAdLoaded()
Log.d(TAG,"adListener.onAdLoaded")
}
override fun onAdFailed(errorCode: Int) {
Log.e(TAG,"NativeAd.onAdFailed() with errorCode $errorCode")
}
override fun onAdClosed() {
super.onAdClosed()
Log.d(TAG,"adListener.onAdClosed")
}
override fun onAdClicked() {
Log.d(TAG,"adListener.onAdClicked")
super.onAdClicked()
}
override fun onAdOpened() {
Log.d(TAG,"adListener.onAdOpened")
super.onAdOpened()
}
}
4.2. Locate following line to Initialize VideoLifecycleListener
// TODO : Initialize VideoLifecycleListener
videoLifecycleListener = object : VideoLifecycleListener() {
override fun onVideoStart() {
Log.d(TAG,"videoOperator.onVideoStart() : Video playback state: starting to be played "
)
}
override fun onVideoPlay() {
Log.d(TAG,"videoOperator.onVideoStart() : Video playback state: being played ")
}
override fun onVideoEnd() {
Log.d(TAG,"videoOperator.onVideoStart() : Video playback state: playback completed.")
// If there is a video, load a new native ad only after video playback is complete.
}
}
5. Locate following line to call load ads whatever type you want with ad id
// TODO : call load ads with ad id
loadAd(getString(R.string.ad_id_native_video), videoNativeView)
loadAd(getString(R.string.ad_id_native_small), smallNativeView)
loadAd(getString(R.string.ad_id_native), largeNativeView)
6. Locate following line to initialize NativeAdLoader and nativeAd
// TODO : initialize NativeAdLoader and nativeAd
// demo method can use with these params : (adId: String, nativeView: NativeView)
val builder = NativeAdLoader.Builder(this, adId)
builder.setNativeAdLoadedListener { nativeAd ->
Log.d(TAG,"onNativeAdLoaded : Ad Loaded successfully.")
// Display native ad.
showNativeAd(nativeAd, nativeView)
nativeAd.setDislikeAdListener {
Log.d(TAG, "setDislikeAdListener : Ad is closed!")
}
}.setAdListener(adListener)
val adConfiguration = NativeAdConfiguration.Builder()
.setChoicesPosition(NativeAdConfiguration.ChoicesPosition.TOP_RIGHT) // Set custom attributes.
.build()
val nativeAdLoader = builder.setNativeAdOptions(adConfiguration)build()
Log.d(TAG,"loadAd() : nativeAdLoader.loadAd() ")
nativeAdLoader.loadAd(AdParam.Builder().build())
7. Locate following line to create and display NativeAd
// TODO : create and display NativeAd
// demo method can use with these params : (nativeAd: NativeAd, nativeView: NativeView)
// Register a native ad material view.
nativeView.titleView = nativeView.findViewById(R.id.ad_title)
nativeView.mediaView = nativeView.findViewById< View >(R.id.ad_media) as MediaView
nativeView.adSourceView = nativeView.findViewById(R.id.ad_source)
nativeView.callToActionView = nativeView.findViewById(R.id. ad_call_to_action)
// Populate a native ad material view.
(nativeView.titleView as TextView).text = nativeAd.title
nativeView.mediaView.setMediaContent(nativeAd.mediaContent)
if (null != nativeAd.adSource) {
(nativeView.adSourceView as TextView).text = nativeAd.adSource
}
nativeView.adSourceView.visibility =
if (null != nativeAd.adSource) View.VISIBLE else View.INVISIBLE
if (null != nativeAd.callToAction) {
(nativeView.callToActionView as Button).text = nativeAd.callToAction
}
nativeView.callToActionView.visibility =
if (null != nativeAd.callToAction) View.VISIBLE else View.INVISIBLE
// Obtain a video controller.
val videoOperator = nativeAd.videoOperator
// Check whether a native ad contains video materials.
if (videoOperator.hasVideo()) {
Log.d(TAG,"initNativeAdView() : videoOperator.hasVideo ")
// Add a video lifecycle event listener.
videoOperator.videoLifecycleListener = videoLifecycleListener
}
// Register a native ad object.
nativeView.setNativeAd(nativeAd)
6. The following table lists the standard Native Ad Sizes :
Display Form | Dimensions | Aspect Ratio | Test Ad Slot ID |
Large image with text | 1080 x 607 | 16:9 | testu7m3hc4gvm |
Small image with tex | 225 x 150 | 3:2 | testb65czjivt9 |
Video with text | 640 x 360 | 16:9 | testy63txaom86 |
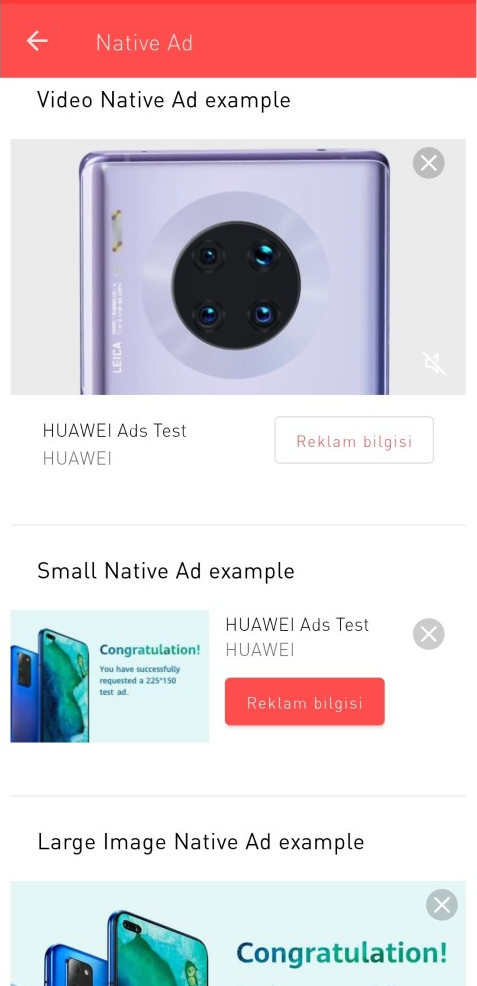
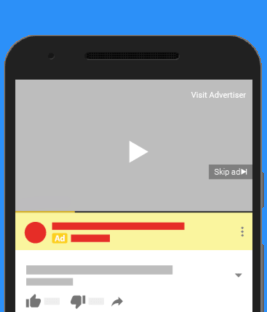
1. Locate following line to add InstreamRollAd layout element InstreamView to XML layout file.
// <!-- TODO : add InstreamView XML element -->
<com.huawei.hms.ads.instreamad.InstreamView
android:id="@+id/instream_view"
android:layout_width="match_parent"
android:layout_height="match_parent" />
2. Locate following line to Initialize NativeView whatever type you want
// TODO : Initialize NativeView
smallNativeView = findViewById(R.id.native_ad_small)
largeNativeView = findViewById(R.id.native_ad_large)
videoNativeView = findViewById(R.id.native_ad_video)
2. Locate following line to create InstreamView, InstreamAd List, InstreamAdLoader, and create Listeners of InstreamAd
// TODO : create InstreamView
private lateinit var instreamView: InstreamView
// TODO : create List< InstreamAd >
private var instreamAds: List< InstreamAd > = ArrayList()
// TODO : create InstreamAdLoader
private lateinit var instreamAdLoader: InstreamAdLoader
// TODO : create InstreamAdLoadListener
private lateinit var instreamAdLoadListener: InstreamAdLoadListener
// TODO : create InstreamMediaChangeListener
private lateinit var instreamMediaChangeListener: InstreamMediaChangeListener
// TODO : create InstreamMediaStateListener
private lateinit var instreamMediaStateListener: InstreamMediaStateListener
// TODO : create InstreamMediaStateListener
private lateinit var mediaMuteListener: MediaMuteListener
4. Locate following line to call AdListeners
// TODO : call createInstreamAdLoadListener
createInstreamAdLoadListener()
// TODO : call createInStreamMediaChangeListener
createInStreamMediaChangeListener()
// TODO : call createInStreamMediaStateListener
createInStreamMediaStateListener()
// TODO : call createMediaMuteListener
createMediaMuteListener()
4.1. Locate following line to Initialize InstreamAdLoadListener
// TODO : Initialize InstreamAdLoadListener
instreamAdLoadListener = object : InstreamAdLoadListener {
override fun onAdLoaded(ads: MutableList< InstreamAd >) {
if (null == ads || ads.size == 0) {
// You can playNormalVideo()
return
}
val it = ads.iterator()
while (it.hasNext()) {
val ad = it.next()
if (ad.isExpired) {
it.remove()
}
}
if (ads.size == 0) {
// You can playNormalVideo()
return
}
loadButton.text = "Ad Loaded"
instreamAds = ads
Log.d(TAG,"onAdLoaded, ad size: " + ads.size + ", you can PlayInStreamAd.")
)
// TODO call display InstreamAds
playInstreamAds(instreamAds)
}
override fun onAdFailed(errorCode: Int) {
Log.e(TAG,"instreamAdLoader.onAdFailed() with errorCode $errorCode")
loadButton.text = "Load Ad"
// You can playNormalVideo()
}
}
4.2. Locate following line to Initialize InstreamMediaChangeListener
// TODO : Initialize InstreamMediaChangeListener
instreamMediaChangeListener = InstreamMediaChangeListener { instreamAd -> // Switch from one roll ad to another.
Log.d(TAG,"InstreamMediaChangeListener.onSegmentMediaChange() : InstreamAd : $instreamAd" )
whyThisAdUrl = "null"
whyThisAdUrl = instreamAd.whyThisAd
Log.d(TAG,"InstreamMediaChangeListener.onSegmentMediaChange() : whyThisAd : $whyThisAdUrl")
if (!TextUtils.isEmpty(whyThisAdUrl)) {
whyThisAd.visibility = View.VISIBLE
whyThisAd.setOnClickListener {
startActivity(Intent(Intent.ACTION_VIEW, Uri.parse(whyThisAdUrl)))
}
} else {
whyThisAd.visibility = View.GONE
}
val cta = instreamAd.callToAction
Log.d(TAG,"InstreamMediaChangeListener.onSegmentMediaChange() : callToAction : $cta")
if (!TextUtils.isEmpty(cta)) {
callToAction.visibility = View.VISIBLE
callToAction.text = cta
instreamView.callToActionView = callToAction
}
}
4.3. Locate following line to Initialize InstreamMediaStateListener
// TODO : Initialize InstreamMediaStateListener
instreamMediaStateListener = object : InstreamMediaStateListener {
override fun onMediaProgress(percent: Int, playTime: Int) {
// Playback process.
//Log.d(TAG, "InstreamMediaStateListener.onMediaProgress() : percent : " + percent + " - playTime : " + playTime);
updateCountDown(playTime.toLong())
}
override fun onMediaStart(playTime: Int) {
Log.d(TAG,"InstreamMediaStateListener.onMediaStart() : playTime : $playTime")
updateCountDown(playTime.toLong())
// You can re show create/play Ad Buttons this state
}
override fun onMediaPause(playTime: Int) {
Log.d(TAG,"InstreamMediaStateListener.onMediaPause() : playTime : $playTime")
updateCountDown(playTime.toLong())
}
override fun onMediaStop(playTime: Int) {
// Stop playback.
Log.d(TAG,"InstreamMediaStateListener.onMediaStop() : playTime : $playTime")
updateCountDown(playTime.toLong())
// You can hide create/play Ad Buttons this state
}
override fun onMediaCompletion(playTime: Int) {
// Playback is complete.
Log.d(TAG,"InstreamMediaStateListener.onMediaCompletion() : playTime : $playTime")
updateCountDown(playTime.toLong())
// You can playNormalVideo()
// You can hide create/play Ad Buttons this state
}
override fun onMediaError(playTime: Int, errorCode: Int, extra: Int) {
Log.d(TAG,"InstreamMediaStateListener.onMediaCompletion() : playTime : $playTime")
updateCountDown(playTime.toLong())
}
}
4.4. Locate following line to Initialize InstreamMediaMuteListener
// TODO : Initialize InstreamMediaMuteListener
mediaMuteListener = object : MediaMuteListener {
override fun onMute() {
Log.d(TAG, "MediaMuteListener.onMute()")
isMuted = true
}
override fun onUnmute() {
Log.d(TAG, "MediaMuteListener.onUnmute()")
isMuted = false
}
}
5. Locate following line to call initialize InStreamAdLoader, InstreamAdView and Load InStreamAd
// TODO : call createInStreamAdLoader
createInStreamAdLoader()
// TODO : call initializeInstreamAdView
initializeInstreamAdView()
// TODO : call loadInStreamAd
loadInStreamAd()
5.1. Locate following line to Initialize InstreamAdLoader
// TODO : Initialize InstreamAdLoader
/**
* if the maximum total duration is 60 seconds and the maximum number of roll ads is eight,
* at most four 15-second roll ads or two 30-second roll ads will be returned.
* If the maximum total duration is 120 seconds and the maximum number of roll ads is four,
* no more roll ads will be returned after whichever is reached.
*/
val totalDuration = 60
val maxCountRoll = 4
// "testy3cglm3pj0" is a dedicated test ad slot ID. Before releasing your app, replace the test ad slot ID with the formal one.
val builder = InstreamAdLoader.Builder(applicationContext, getString(R.string.ad_id_roll))
// Before reusing InstreamAdLoader to load ads, ensure that the previous request is complete.
// Set the maximum total duration and number of roll ads that can be loaded.
instreamAdLoader = builder
.setTotalDuration(totalDuration)
.setMaxCount(maxCountRoll)
.setInstreamAdLoadListener(instreamAdLoadListener)
.build()
5.2. Locate following line to Initialize InstreamAdView
// TODO : Initialize InstreamAdView
instreamView = findViewById(R.id.instream_view)
instreamView.setInstreamMediaChangeListener (instreamMediaChangeListener)
instreamView.setInstreamMediaStateListener (instreamMediaStateListener)
instreamView.setMediaMuteListener(mediaMuteListener)
instreamView.setOnInstreamAdClickListener {
Log.d(TAG,"setOnInstreamAdClickListener")
}
5.3. Locate following line to load InStreamAd
// TODO : load InStreamAd
if (null != instreamAdLoader) {
loadButton.text = "Loading"
instreamContainer.visibility = View.VISIBLE
// you can customize ad RequestOptions parameters
instreamAdLoader.loadAd(AdParam.Builder().build())
} else {
Log.d(TAG,"instreamAdLoader id NULL. You must create Ad before loading Ad")
}
6. Locate following line to display InStreamAd
// TODO : display InStreamAd
maxAdDuration = getMaxInstreamDuration(ads)
instreamContainer.visibility = View.VISIBLE
loadButton.text = "Load Ad"
instreamView.setInstreamAds(ads)
// You can re show create/play Ad Buttons this state
7. Locate following line to play or pause InStreamAd
// TODO : play or pause InStreamAd
if (instreamView.isPlaying) {
instreamView.pause()
pauseButton.text = "Play"
} else {
instreamView.play()
pauseButton.text = "Pause"
}
// You can use in onResume, onPause methods
7. Locate following line to mute or unMute InStreamAd
// TODO : play or pause InStreamAd
// isMuted veriable is updating in mediaMuteListener onMute/onUnmute methods
if (isMuted) {
instreamView.unmute()
muteButton.text = "Mute"
} else {
instreamView.mute()
muteButton.text = "UnMute"
}
8. Locate following line to skipAd InStreamAd
// TODO : skipAd InStreamAd
skipAd.setOnClickListener {
if (null != instreamView) {
instreamView.onClose();
instreamView.destroy();
instreamContainer.removeView(instreamView);
instreamContainer.setVisibility(View.GONE);
instreamAds.clear();
//Log.d(TAG, "skipped Ad, and you need new Ad load by start first process.");
}
}
6. The following table lists the standard InstreamRoll Ad Sizes :
Display Form | Dimensions | Test Ad Slot ID |
Image or video | 640 x 360 | testy3cglm3pj0 |
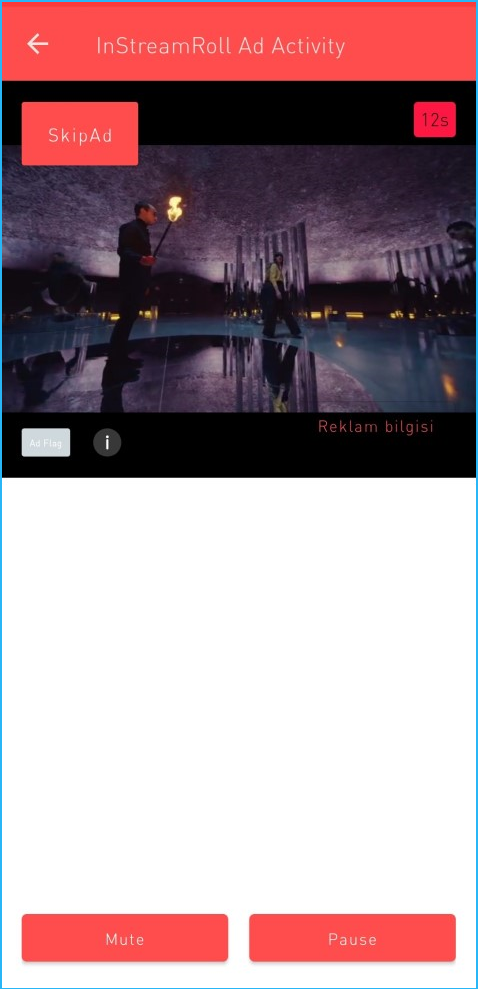
Test and Verification
Upon completing the essential parts of the code, connect your mobile device to the PC and enable the USB debugging mode. In the Android Studio window, click Run icon to run the project you have created in Android Studio to generate an APK. Then install the APK on the mobile device.
- Open the app upon installing it to your device
- Click the Buttons that is identified by Ad types.
- Examine usage of ad types, impressions and customization details
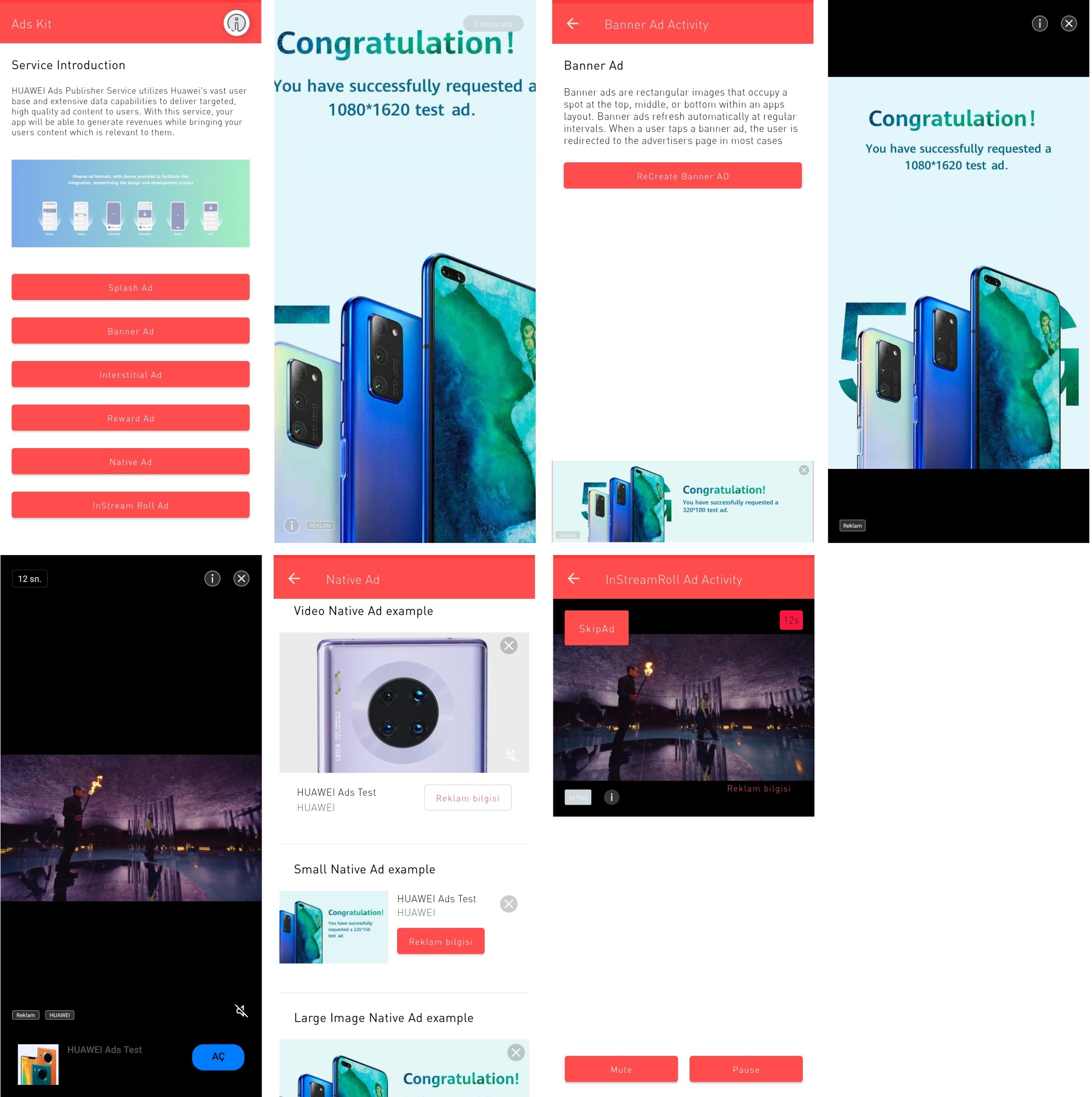
Well done. You have successfully completed this Codelab and learned:
- How to developt app with Kotlin lanugage
- How to use Huaewi Ads Kit SDK
- How to use SplashAd
- How to use BannerAd
- How to use InterstialAd
- How to use RewardAd
- How to use NativeAd
- How to use InStreamRollAd